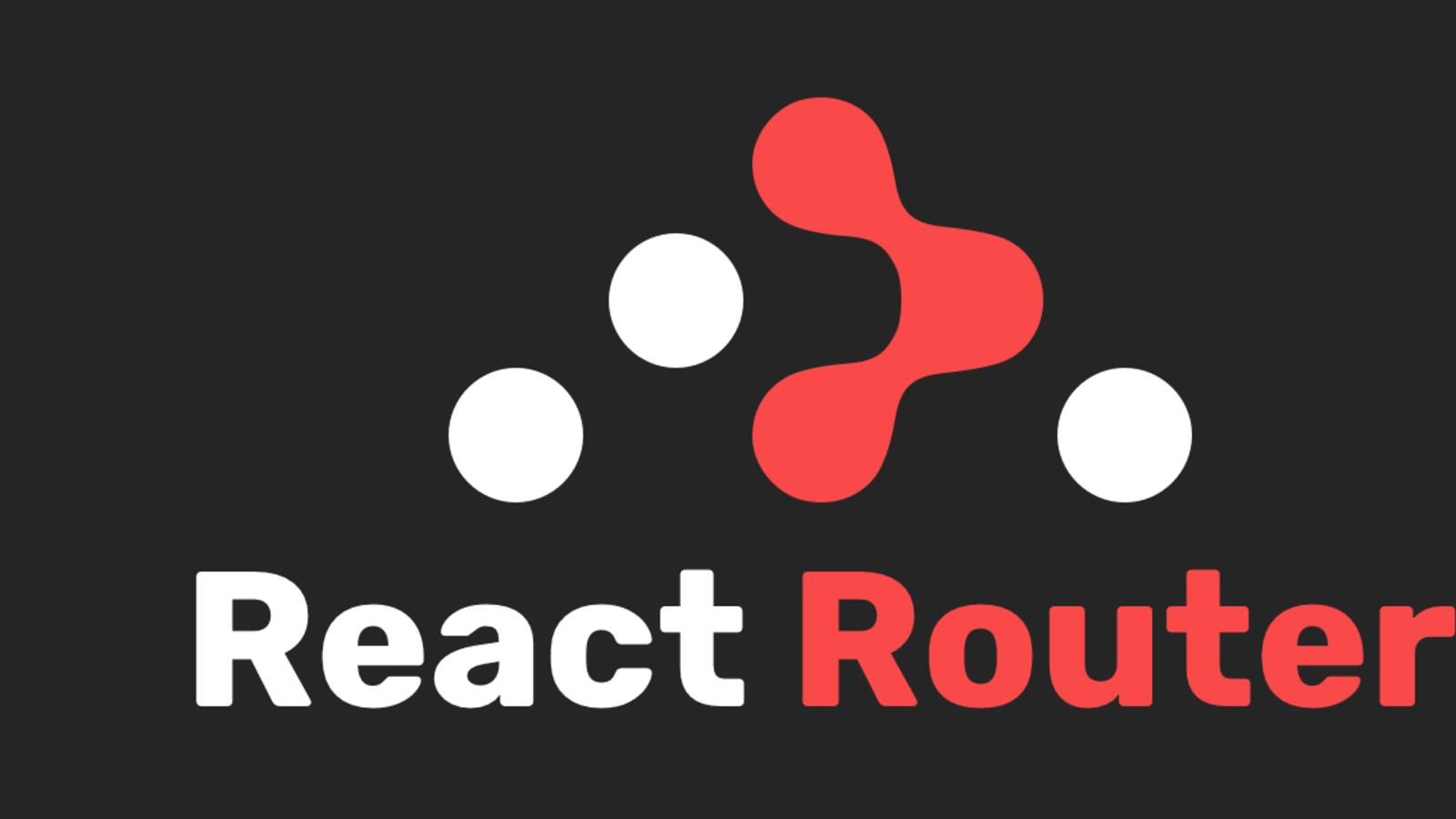
Demystifying React Router: Navigating Your Single-Page Applications
-
Arnaud Fernandés
- November 2, 2023
- 02 Mins read
- #Development #React
Introduction
React Router is a powerful routing library for React applications that enables developers to manage navigation and URL changes in single-page applications (SPAs). It provides a seamless way to create dynamic, interactive user interfaces without the need for full page reloads. In this article, we’ll delve into the world of React Router, exploring its core concepts and demonstrating how to leverage it effectively in your projects.
Understanding Single-Page Applications (SPAs)
Before diving into React Router, it’s essential to grasp the concept of single-page applications. Unlike traditional multi-page applications, SPAs load a single HTML page and dynamically update its content as the user interacts with the application. This creates a smoother, more responsive user experience.
Installing React Router
Getting started with React Router is straightforward. You can install it via npm by running the following command:
npm install react-router-dom
Basic Usage
1. Setting Up Routes
Define the routes in your application using BrowserRouter
and Route
components from react-router-dom
. Routes are defined within your application’s entry point, typically in the App.js
or a similar file.
import { BrowserRouter as Router, Route } from "react-router-dom";
const App = () => {
return (
<Router>
<div>
<Route path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</div>
</Router>
);
};
2. Creating Components
Create the corresponding components for each route (e.g., Home
, About
, Contact
). These components will be rendered when the associated route is matched.
const Home = () => <div>Home Page</div>;
const About = () => <div>About Page</div>;
const Contact = () => <div>Contact Page</div>;
3. Navigating Between Routes
Utilize Link
components to create navigation links within your application.
import { Link } from "react-router-dom";
const Navigation = () => {
return (
<div>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
<Link to="/contact">Contact</Link>
</div>
);
};
Route Parameters and Dynamic Routes
React Router allows for dynamic routing by using route parameters. These parameters are placeholders in the URL that can be extracted and used to fetch specific data or render dynamic content.
<Route path="/user/:id" component={UserDetail} />
In this example, :id
is a route parameter that can be accessed in the UserDetail
component.
Nested Routes
React Router also supports nested routes, allowing you to create complex UI structures with different levels of routing.
<Route path="/products" component={Products}>
<Route path="/products/:id" component={ProductDetail} />
</Route>
Conclusion
React Router is an essential tool for building dynamic, interactive single-page applications. By effectively managing navigation and URL changes, it enables developers to create seamless user experiences. With features like route parameters and nested routes, React Router provides the flexibility needed to handle a wide range of application scenarios.
Remember, this article only scratches the surface of what React Router can do. As you delve deeper into your projects, you’ll discover even more powerful capabilities it offers.
For detailed documentation and advanced usage, refer to the official React Router documentation.
Happy routing!
Note: The examples provided in this article assume a basic understanding of React. If you’re new to React, consider reviewing the official React documentation for a solid foundation.